Introduction
Bash scripting serves as a valuable utility for modern and comprehensive task in administration OS like Linux and macOS. Its straightforward syntax, close integration with the operating system, and efficiency in handling shell—specific functionalities provide distinct advantages for specific use cases.
Advantages of Bash Scripting
- Streamlined Automation: Bash scripts offer a lightweight and intuitive approach to automate repetitive tasks and manage system administration responsibilities. They swiftly execute sequences of commands, optimizing tasks such as backups, log management, and user configuration;
- Seamless System Integration: As the default shell on most Unix—like systems, Bash seamlessly integrates with the underlying OS. This tight integration grants easy access to system utilities, environment variables, and system information, streamlining operations that involve system—related tasks;
- Command—Line Efficiency: Bash excels at handling the command—line interface, making it proficient in tasks like text manipulation, file processing, and directory navigation. Its support for pipes, redirects, and other shell—specific features further enhances its capabilities;
- Rapid Prototyping and Testing: With its simple syntax and immediate feedback during command—line execution, Bash facilitates rapid prototyping and testing of ideas and solutions. This agility proves valuable for quick fixes and temporary scripts;
- Accessible for Sysadmins: Bash scripts are accessible to system administrators, even without extensive programming knowledge. This accessibility empowers sysadmins to craft custom solutions and automate tasks with ease;
- Optimal Shell Built—ins: Bash provides built—in commands that are highly optimized for performance, rendering it efficient for small—scale and repetitive tasks. This feature allows Bash scripts to execute certain operations faster compared to higher—level programming languages;
- Platform Portability: Although primarily used on Unix—like systems, Bash is available on various platforms, including Linux, macOS, and Windows (via Cygwin or Windows Subsystem for Linux). This portability enables Bash scripts to be utilized across diverse environments.
In conclusion, Bash scripting's strengths lie in its simplicity, tight integration with Unix—based systems, and proficiency in handling shell—specific tasks. It excels in automating system administration tasks, working seamlessly with command—line utilities, and providing quick solutions for text processing and file manipulation. Its accessibility and ability to swiftly prototype ideas make it an excellent choice for sysadmins and tasks that require a concise and effective scripting approach.
Requirements
- Root rights;
- Debian 11 or higher version;
- Several knowledge about work OS ;
- Internet connection.
Start your engine
We will work in VPS machine by our provider Serverspace, which you can order on the site. And use Debian 11 operating system with bash shell. Firts of all go to the control panel of Serverspace and choose needed platform to work:
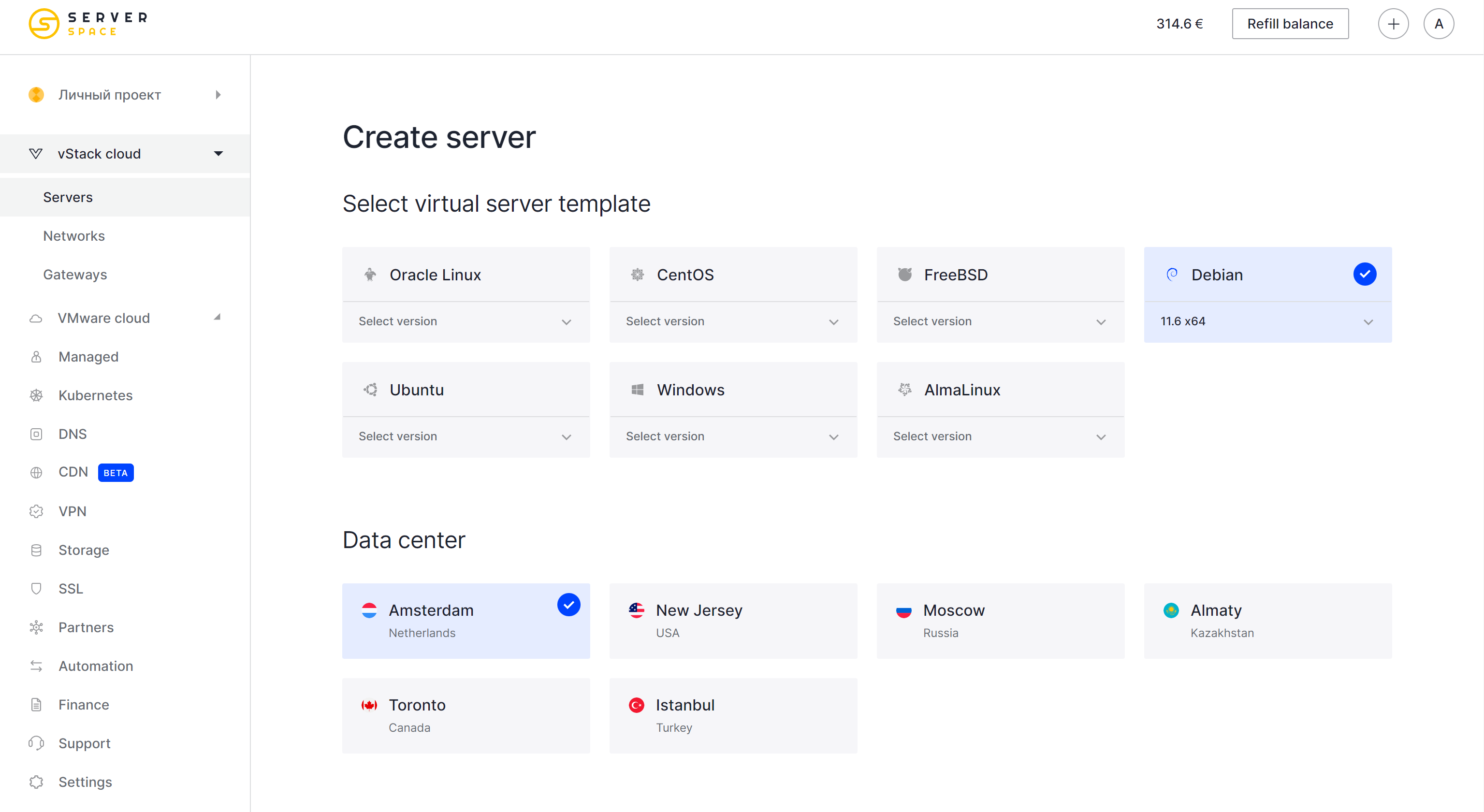
You can see various of data—centers in different countries, choose at your point of view and purposes, preferably near to your location. Then you can look at operating system and choose one, for our example we haved look classic.
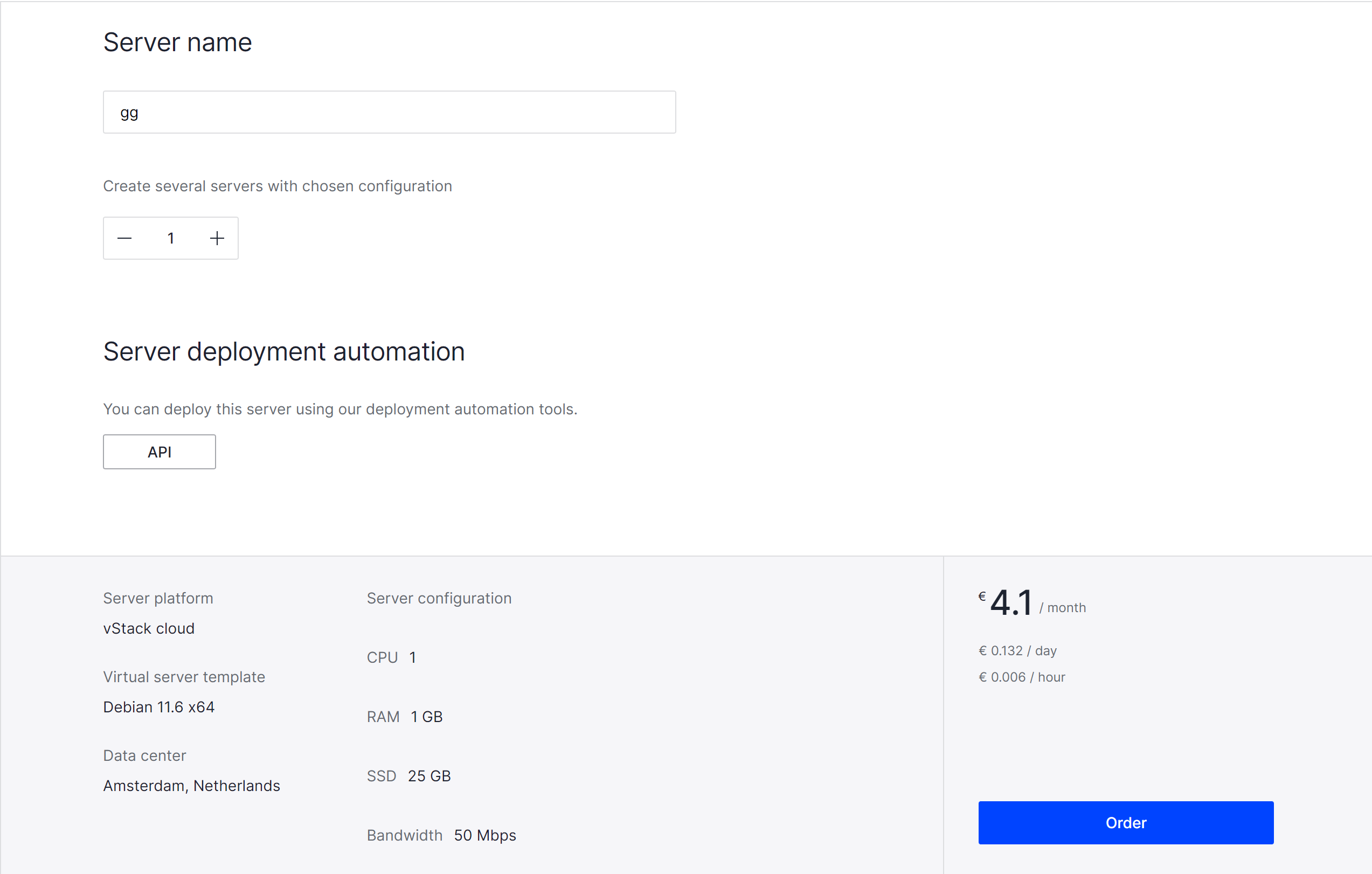
After choice of resources for your machine you can see the price and click on Order button, wait to finished setup server. After that enter your credentials to login into the system via command bellow.
ssh root@162.64.253.1
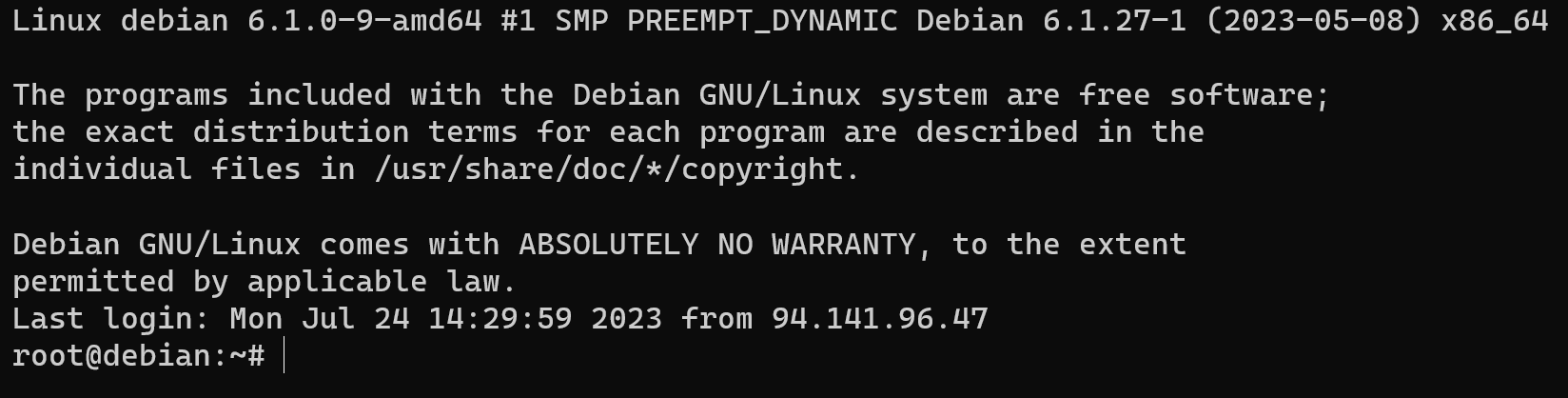
Reminder! You have to replace for your IP—address and enter password at the account.
Create firts script
Congratulations, we are in. And already used bash interpreter and shell. The sign # or if you login by user $ that means the machine already to read and process entering command in bash. At second, we install text editor for more comfortable usage our script file:
apt install nano
You can also use more easily and more complexity text editors like: gedit, vim, Emacs, etc. Choose what you want for your comfortable work. Let's create our firts script. For that you have to find path to bash in your system, at the default it's /bin/bash, but you can find it by command:
cd / && which bash
Next step will create a script file by the command below:
nano /etc/script.sh
And enter this lines:
#!/bin/bash
echo "My first script in bash!"

In the first line we indicate interpreter for script, write your path as we known above, but in 99% cases we have default path. On the line below we can see command echo which displayed at the screen our text. Correct mod to executable:
cd etc && chmod +x script.sh
Now you can start your script by the path of file or using command bellow:
sh script.sh

Here we go! We have first wrote script, but look at the more complexity and useful detail of scripting process.
Arguments
There is input data which need to process by the script, data which entering by the user or transfer by another utility, service or script. For that in programming language there is definition — arguments or data needed to process by algorithm. Let's have a look at the example:
For that create new file and open by the one command:
nano /etc/price.sh && chmod +x /etc/price.sh
Write script below:
#!/bin/bash
echo "My suggested price is $1"
Explanation: In that script we use argument and indicate this by sign $K, where does K be a number of entering argument. The $0 always is name of running script, $1, $2 and etc. It's just a list of entering data. Let's run it!
sh /etc/price.sh 4333$
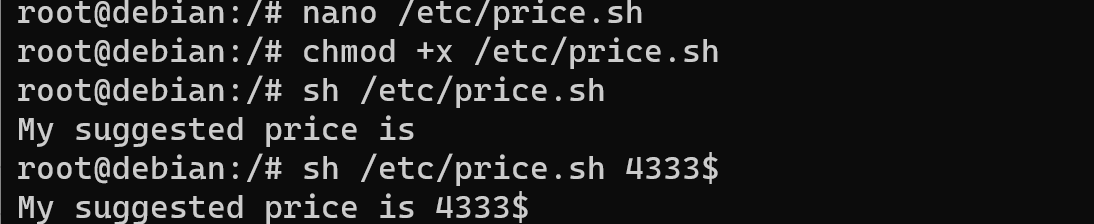
You may notice empty part of displayed raw, that has happened due to we are not provide for an error and have a bug! Let's make check mechanism:
nano /etc/price.sh
And enter that code:
#!/bin/bash
if [ "$1" -z]; then
echo "You entering data don't accordance rule!"
exit 1
fi
echo "My suggested price is $1"
Explanation: we add function of checking entering data by if—then—else construction. If meaning syntax for start condition command, which we write and indicate filter for variable. In that case we use —z option, which means that field is empty. Next we wrote then command which indicate action if statement above was true. In our case we displayed error message and use exit 1 — command for finished program and indicate error. Let's start and check:
sh /etc/price.sh 44$ && sh /etc/price.sh

Alright! But if we need to use prepare data in the script? For that purpose we have variable!
Variables
They represent cell of storage, which save information and allow to use that by mention it in script. Variable Declaration: Variables in Bash are declared without using keywords, simply assigning a value to a variable. For example:
name="John" age=30
Variables in Bash support a variety of data types thanks to dynamic typing. Variable names must start with a letter or the symbol "_", letters, numbers are allowed. Make it easier to understand the code by using meaningful variable names, and use the dollar symbol "$" to access their values, for example:
echo "Hello, $name!"
The value of the variable can be reassigned, changed at any time of script execution:
count=5
echo "Current value of count: $count"
count=10
echo "New value of count: $count"
Reading a value from a user: Using the read command, you can read a value from a user and assign it to a variable:
read -p "Enter your price: " price
echo "Awwww, $price?"
Deleting a variable: To delete a variable, use the unset command:
unset name
Exporting a variable: If a variable needs to be made available to subprocesses, you can use the export command:
export MY_VARIABLE="price"
Variables in Bash play an important role when writing scripts and automating tasks, allowing you to store and process data throughout the execution of a program or commands.
Control structures
In any script there is logical path which information go through, filtering, changing value and give a result. All of that can possible due to control structures, which adding branches and capability of choice. There is cycles, conditional constructions, etc. Simple example we explain above about if—then—else constrution. That commonly used for single or two—three branching and used structure:
if [ condition ]; then
statement-1
elif [ condition-2 ]; then
statement-2
fi
In that example we see 3 branches, but what do we need do if we have list of conditions and one parameter? For that we can use switch—case construction. All meaning include in our parameter compare to other in list, therefore execute action accordance to reply. For example:
read -p "What is machine you search for? " car
case "$car" in
"Volkswagen" )
price="3000$"
;;
"BMW" )
price="4000$"
;;
*)
price="we don't know"
esac
echo "You requesting cost of car: $price"
Enter that in file as we make previous before! And run it:
./etc/price.sh
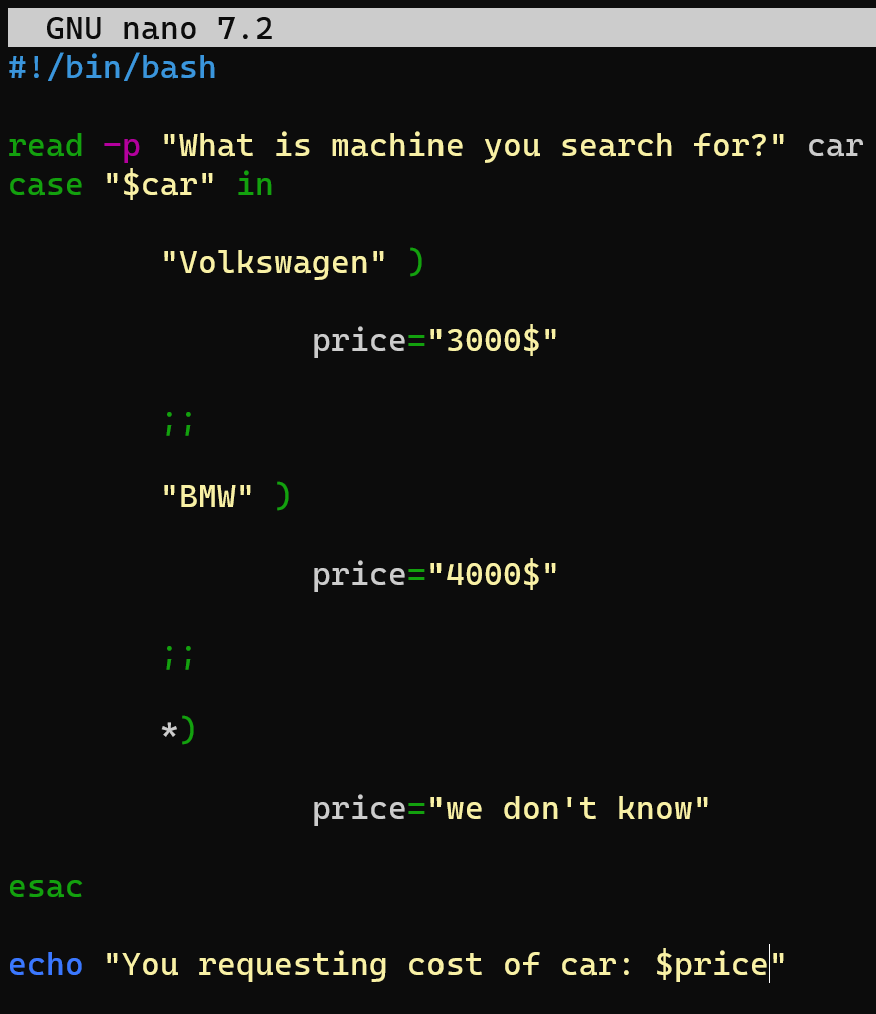
After start let's check:
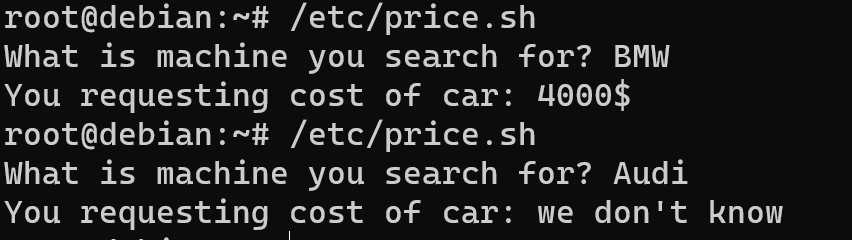
Alright, that work! There is next problem, some commands we need to execute certain number of times or until conditions will complete. For that we use cycles!
Cycles
We can use cycle for which allows us to iterate certain command for each object in sequence, for example:
cars=("Toyota" "Honda" "Ford" "BMW" "Tesla")
for ((i=0; i<${#cars[@]}; i++))
do
echo "Car $((i+1)): ${cars[i]}"
done
That code allow us to iterate indicate value certain number of times. i=0 syntax indicate start value, i<${#cars[@]} it's condition, i++ it's command if condition accordance logical value true. Then do it's command to do after completion condition in our example it's echo and done means finished cycle. Let's test:
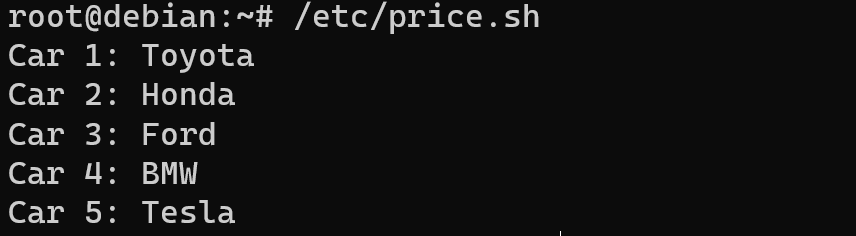
The while cycle in the example:
cars=("Toyota" "Honda" "Ford" "BMW" "Tesla")
count=1
while [ $count -le ${#cars[@]} ]
do
echo "Car $count: ${cars[$((count-1))]}"
((count++))
done
Conclusion
Bash scripting sets itself apart with its user—friendly nature, smooth integration into Unix—based systems, and adeptness in handling shell—specific tasks. It shines in automating system administration tasks, seamlessly interacting with command—line utilities, and swiftly delivering solutions for text processing and file manipulation. Its accessibility and flexibility make it the preferred option for sysadmins tackling tasks that require a concise and efficient scripting approach. Proficiency in Bash scripting empowers administrators to boost productivity, streamline task management, and unlock the full capabilities of Linux and macOS systems, culminating in a more efficient and productive computing environment.